React Components & Reusability: How We Build Scalable Websites and MVPs
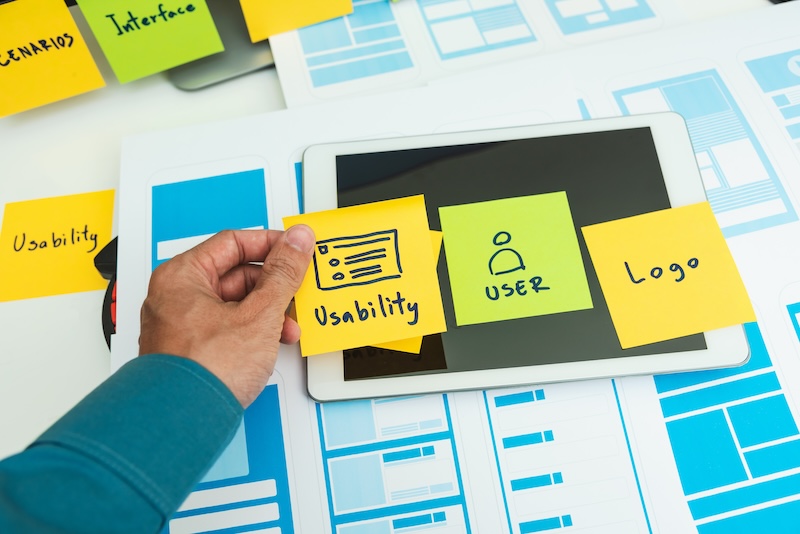
In modern web development, building scalable and maintainable applications is essential for growth. React, a popular JavaScript library, has transformed how we approach development through its component-based structure. By designing reusable components, developers can streamline workflows, improve maintainability, and create scalable websites and Minimum Viable Products (MVPs). In this article, we’ll explore how React’s reusability features enable us to build efficient, high-performance applications tailored to evolving business needs.
1. Why Reusability Matters in Web Development
Reusability is the principle of designing components that can be used multiple times across different parts of an application. By creating reusable components, developers reduce redundancy, save time, and improve consistency. In the context of React, reusability is a cornerstone of the library’s architecture, allowing teams to build scalable applications that are easier to maintain and expand.
Benefits of Reusable Components
- Efficiency: Reusable components streamline development by reducing the need to rewrite code for similar features.
- Consistency: With reusable components, developers can maintain a consistent look and feel across an application, enhancing user experience.
- Scalability: Reusable components simplify scaling, as new features can be integrated without extensive changes to the existing codebase.
- Maintainability: Reusable components promote modular code, making it easier to locate, update, and debug specific functionalities.
The reusability of components empowers teams to build applications that are adaptable and efficient, ensuring long-term scalability.
2. Understanding React’s Component-Based Architecture
React is built on a component-based architecture, which means that the user interface is broken down into smaller, independent, and reusable pieces called components. Each component encapsulates its structure, logic, and styling, allowing it to function independently within the application.
Types of React Components
-
Functional Components: These are simpler components defined as JavaScript functions. They accept props as arguments and return JSX, making them ideal for creating reusable UI elements.
-
Class Components: These components are defined using JavaScript classes. While they offer more control over lifecycle methods, functional components with hooks are increasingly preferred due to their simplicity.
-
Higher-Order Components (HOCs): HOCs are functions that take a component as an argument and return a new component. They enable code reuse by encapsulating logic that can be shared across multiple components.
-
Compound Components: Compound components are a design pattern that allows components to work together as a group. This approach is useful for building complex, reusable UI elements like dropdowns and modals.
Understanding these component types and their applications is key to building scalable React applications.
3. Building Reusable Components: Key Principles
Creating truly reusable components requires careful planning and adherence to specific design principles. Below are some best practices for building reusable React components.
Focus on Single Responsibility
Each component should have a single responsibility, meaning it should focus on one specific task. By limiting a component’s functionality to a single purpose, developers ensure that the component remains focused, modular, and reusable. This principle also enhances readability, as each component’s purpose is clear.
Use Props for Flexibility
Props are React’s way of passing data from parent to child components. By using props, developers can create flexible components that adapt to different use cases. For example, a button component could receive props for label text, color, and onClick functionality, allowing it to serve various purposes across an application.
Avoid Hardcoding and Use Configurations
Hardcoding values within components limits their flexibility. Instead, use props or configuration objects to make components customizable. For instance, avoid hardcoding styles or text and instead pass them as props so that the component can adapt to different requirements.
Compose Components Using Composition Patterns
Component composition is the process of combining components to create more complex UI structures. React encourages composition over inheritance, allowing developers to build rich, complex interfaces by composing smaller components together.
4. Leveraging React Hooks for Reusability
With the introduction of React Hooks, functional components gained more flexibility, enabling developers to use state and lifecycle methods. Hooks have revolutionized how we build reusable components in React by encapsulating logic and state within functional components.
Common Hooks for Reusability
- useState: Manages local component state, making components dynamic and interactive.
- useEffect: Handles side effects, such as data fetching and DOM manipulation, allowing developers to control component behavior based on lifecycle events.
- useContext: Shares data across components without passing props manually through each level, promoting data consistency.
- Custom Hooks: Custom hooks are user-defined functions that encapsulate reusable logic, making it easy to reuse complex stateful behavior across different components.
By utilizing hooks, developers can achieve greater reusability, encapsulating behavior in a way that’s easy to apply across multiple components.
5. Case Study: Building Scalable MVPs with Reusable Components
Let’s look at how Prompt Launch leveraged React’s reusability features to build an MVP for a client in the e-commerce industry. The client needed an MVP with scalable features, including a product listing page, search functionality, and a shopping cart.
Step 1: Designing Reusable UI Components
We started by designing a set of reusable UI components, including buttons, form fields, and product cards. Each component was built with props to handle variations in style and functionality, ensuring that they could be reused across different pages.
Example: Product Card Component
The product card component was designed to display essential product details like image, name, and price. By using props for the product data, we ensured that the component could dynamically render different products without modification.
Step 2: Using Context for State Management
We used React’s Context API to manage global state, such as the shopping cart and user preferences. By storing these values in a context provider, we avoided prop-drilling, making it easier to manage state and ensuring consistency across components.
Step 3: Implementing Custom Hooks
To handle complex functionality like data fetching and form validation, we created custom hooks. For instance, a custom hook called useFetchProducts
was used to fetch and filter product data, allowing the component to access data without repetitive code.
The result was an MVP that met the client’s requirements and offered scalable, reusable components that could be extended as the product evolved.
6. Best Practices for Reusability and Performance Optimization
To build highly performant React applications, it’s important to follow best practices for reusability and optimization. Here are some strategies we implement at Prompt Launch.
Optimize Component Rerenders
Rerendering can impact performance, especially in large applications. Techniques like React.memo
and useMemo
help optimize performance by preventing unnecessary rerenders. React.memo
memorizes a component, while useMemo
caches values, reducing redundant calculations.
Use Lazy Loading and Code Splitting
Lazy loading allows components to load only when needed, reducing initial load time. Code splitting with tools like Webpack or libraries like React’s React.lazy
improves application performance by breaking the app into smaller chunks.
Avoid Prop Drilling
Prop drilling occurs when props are passed through multiple levels of components, which can become difficult to manage. Instead, use context or state management libraries like Redux to handle data more efficiently, ensuring reusability without clutter.
Test Components Independently
Reusable components should be independently tested to ensure they function correctly in different contexts. Tools like Jest and React Testing Library enable developers to create unit tests that verify each component’s behavior, reducing the likelihood of bugs and ensuring reliability.
7. Conclusion: React Components for Scalable Web Development
React’s component-based architecture, combined with hooks and reusability best practices, provides a powerful foundation for building scalable, maintainable websites and MVPs. By focusing on single responsibility, using props for flexibility, and optimizing performance, developers can create reusable components that meet the demands of dynamic, modern applications.
At Prompt Launch, we leverage React’s capabilities to design components that streamline development, enhance maintainability, and ensure scalability. Ready to build a scalable MVP with React? Contact Prompt Launch to create a high-quality, future-proof application tailored to your business needs.