How Next.js Enhances SEO for Single Page Applications (SPAs)
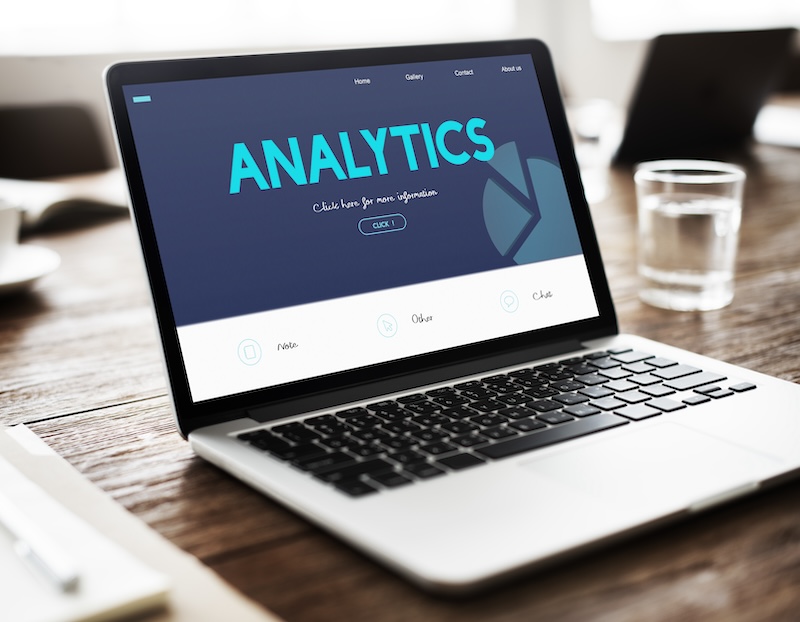
Single Page Applications (SPAs) offer a seamless user experience by dynamically updating content without refreshing the entire page. While SPAs provide a fast and fluid experience, they face unique challenges when it comes to SEO. Traditional SPAs, often built with frameworks like React, load content on the client side, making it difficult for search engines to crawl and index. Next.js, a popular React framework, solves these issues by providing server-side rendering (SSR), static site generation (SSG), and other SEO-enhancing features.
In this article, we’ll explore how Next.js addresses the SEO challenges of SPAs, making it a powerful tool for building SEO-friendly, high-performance web applications.
1. Understanding the SEO Challenges of Single Page Applications
SPAs deliver content dynamically, allowing users to interact with the page without reloading. However, this client-side rendering approach can limit SEO visibility. Search engines rely on HTML content to crawl and index pages effectively, and SPAs often lack pre-rendered HTML, resulting in SEO challenges.
Key SEO Challenges of SPAs
- Incomplete Content for Crawlers: Client-side rendering can delay content loading, making it difficult for search engine bots to access and index all relevant information.
- Single URL Structure: SPAs typically use a single URL for multiple views, limiting the use of unique URLs that are essential for indexing different sections of the site.
- Dynamic Metadata: Metadata, such as titles and descriptions, often changes dynamically in SPAs. Without pre-rendering, these changes may not be visible to search engines, impacting SEO.
Next.js provides solutions to these challenges by combining server-side rendering, static generation, and advanced routing, making SPAs more accessible and SEO-friendly.
2. How Next.js Improves SEO for SPAs
Next.js enhances SEO for SPAs by offering features that address the limitations of client-side rendering. Its capabilities in server-side rendering, static site generation, and customizable metadata make it an ideal choice for developers looking to optimize SPAs for search engines.
Server-Side Rendering (SSR)
With SSR, pages are rendered on the server at request time and then sent to the client with fully populated HTML content. This approach ensures that search engines can access and index the content, improving SEO visibility.
- Improved Indexing: SSR delivers fully rendered pages to search engines, making it easier for bots to index all content.
- Faster Initial Load: SSR reduces the time it takes for users to see the main content, providing a better user experience and reducing bounce rates.
In Next.js, SSR is easily implemented by using the getServerSideProps
function, allowing developers to retrieve data on the server before rendering the page.
Static Site Generation (SSG)
SSG is another powerful feature of Next.js, allowing pages to be pre-rendered at build time. These pre-rendered pages load instantly, providing both speed and SEO benefits.
- Enhanced Performance: SSG delivers static HTML, resulting in faster load times, especially on mobile devices.
- Better SEO: Since pages are fully rendered and stored as static HTML, search engines can easily access and index content.
Developers can use getStaticProps
in Next.js to implement SSG, making it an ideal solution for pages that don’t need frequent updates.
3. Optimizing Metadata with Next.js
Metadata, including title tags, descriptions, and Open Graph tags, plays a critical role in SEO. With Next.js, developers can dynamically manage metadata on each page, ensuring that each view has relevant SEO information.
Using the Next.js Head Component
The Next.js <Head>
component allows developers to set page-specific metadata for each route. By customizing titles, descriptions, and social sharing tags, developers can optimize each page for search engines and improve click-through rates.
import Head from "next/head";
function MyPage() {
return (
<div>
<Head>
<title>My Awesome Page</title>
<meta
name="description"
content="This is a description for SEO purposes."
/>
</Head>
<h1>Welcome to My Awesome Page</h1>
</div>
);
}
Dynamic Metadata for Enhanced SEO
For applications with dynamic content, metadata can be set programmatically based on page data. This allows developers to customize metadata for each product, article, or category, ensuring that every page is optimized for SEO.
With Next.js, managing dynamic metadata is straightforward, enabling developers to maintain SEO standards across different pages.
4. Advanced Routing for Unique URLs
Unique URLs are essential for SEO, as they allow search engines to index individual pages. Next.js provides an advanced routing system that supports dynamic routes, making it possible to create SEO-friendly URLs for each view.
Dynamic Routing in Next.js
Next.js’s file-based routing enables developers to create dynamic routes that adapt to content. For example, a blog with multiple articles can use dynamic routing to generate unique URLs for each article, such as /blog/my-awesome-article, enhancing SEO by enabling search engines to index individual posts.
import { useRouter } from "next/router";
function BlogPost() {
const router = useRouter();
const { slug } = router.query;
return <h1>Blog Post: {slug}</h1>;
}
export default BlogPost;
Dynamic routing allows developers to structure URLs in a way that is both user-friendly and SEO-friendly, improving the site’s overall searchability.
5. Leveraging Image Optimization in Next.js
Images play a crucial role in user experience and SEO. However, large, unoptimized images can slow down a website, negatively affecting SEO. Next.js offers built-in image optimization features to enhance website performance and SEO.
Next.js Image Component
The Next.js component automatically optimizes images by resizing, compressing, and serving them in next-gen formats like WebP. This optimization reduces load times and improves Core Web Vitals, which are essential for SEO.
import Image from "next/image";
function MyComponent() {
return (
<Image
src="/my-image.jpg"
alt="An optimized image"
width={500}
height={300}
/>
);
}
By optimizing images, Next.js ensures that SPAs load quickly and provide a smooth experience, contributing to better SEO performance.
6. Core Web Vitals and Performance Optimization in Next.js
Google’s Core Web Vitals are performance metrics that assess loading speed, interactivity, and visual stability. Next.js is optimized to help developers meet Core Web Vitals, enhancing SEO and user experience.
Optimizations for Largest Contentful Paint (LCP)
LCP measures the time it takes for the main content to load. Next.js improves LCP through SSR, SSG, and image optimization, reducing load times and improving search rankings.
Reducing Cumulative Layout Shift (CLS)
CLS measures visual stability. By reserving space for images and other media, Next.js prevents layout shifts, ensuring a stable visual experience for users and enhancing SEO.
Improving First Input Delay (FID)
FID measures responsiveness. Next.js optimizes FID by enabling efficient code-splitting and reducing JavaScript bundle sizes, making interactions smoother and faster.
These optimizations align with Core Web Vitals, helping SPAs achieve better performance and SEO outcomes.
7. Case Study: Using Next.js to Optimize an SPA for SEO
Prompt Launch recently partnered with a client to improve their SEO through Next.js. The client’s SPA faced challenges with slow load times, low search rankings, and poor mobile performance. By implementing Next.js, we transformed their website’s SEO and performance.
Solutions Implemented
- SSR and SSG: We used SSR and SSG to pre-render pages, ensuring that search engines could access and index all content.
- Dynamic Metadata: Metadata for each page was dynamically set to improve visibility and click-through rates.
- Image Optimization: Images were optimized using Next.js’s Image component, improving load times and Core Web Vitals scores.
Results
- Higher Search Rankings: Improved visibility and rankings for targeted keywords.
- Reduced Load Times: Load times were cut in half, improving user retention.
- Increased Organic Traffic: The client saw a 40% increase in organic traffic due to better search engine visibility.
- This case study demonstrates how Next.js can transform an SPA into an SEO-friendly, high-performance website.
8. Conclusion: Next.js as an SEO Solution for SPAs
Next.js addresses the SEO challenges of SPAs by providing server-side rendering, static site generation, dynamic metadata management, and performance optimizations. By leveraging Next.js, developers can build SPAs that are not only fast and engaging but also SEO-friendly.
If you’re looking to optimize your SPA for search engines, Contact us to explore how Next.js can elevate your website’s SEO and user experience.