Case Study: Building a SaaS MVP with React and Next.js (Part 1)
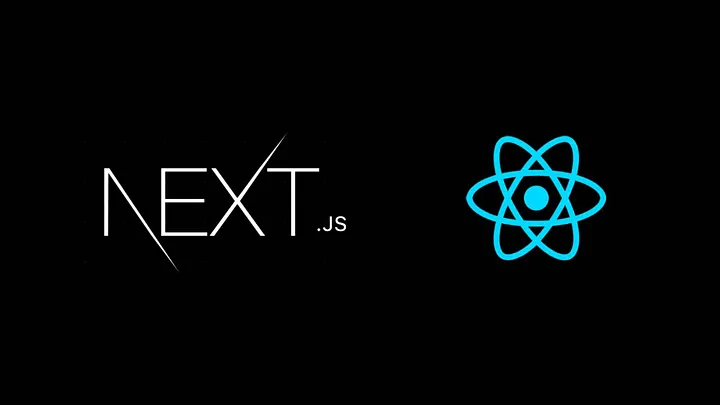
Creating a Minimum Viable Product (MVP) for a Software as a Service (SaaS) application is a journey that requires careful planning, strategic thinking, and technical expertise. This case study explores the step-by-step process of building a SaaS MVP using React and Next.js, highlighting the decisions, challenges, and solutions involved. In this first part, we’ll cover the initial stages, from ideation and planning to setting up the development environment and building the foundational components of the application.
1. Project Overview and Objectives
The goal of this SaaS MVP was to create a platform that streamlined project management for small to mid-sized teams. The application needed to be intuitive, responsive, and scalable, with a focus on core functionalities such as task management, team collaboration, and progress tracking.
Key Objectives
- Quick Launch: Develop a functional MVP within a short timeframe to gather user feedback.
- Core Features: Include essential features to validate the concept, such as task creation, team assignments, and activity tracking.
- Scalability: Build a foundation that could support future enhancements without extensive rewrites.
- Responsive Design: Ensure compatibility across devices to cater to diverse user needs.
By setting clear objectives, we established a roadmap that would guide each phase of the development process.
2. Choosing the Tech Stack: Why React and Next.js?
For this project, we chose React and Next.js as the primary technologies due to their strengths in creating dynamic, SEO-friendly, and scalable applications.
Benefits of React
React’s component-based architecture allows us to build reusable, modular elements, reducing development time and making it easier to maintain the codebase. Additionally, React’s rich ecosystem and active community provided access to a wealth of libraries and tools that accelerated the development process.
Why Next.js?
Next.js offers features like server-side rendering (SSR) and static site generation (SSG), which are essential for building SEO-friendly applications. With SSR, we could render pages on the server, enhancing performance and ensuring that search engines can crawl the content. Next.js also simplifies routing, data fetching, and other aspects of web development, making it an ideal choice for a SaaS MVP.
3. Planning and Defining Core Features
In the planning phase, we worked closely with stakeholders to outline the core features required for the MVP. Our goal was to focus on the “must-have” features that would provide value to users while keeping the scope manageable.
Using the MoSCoW Method
To prioritize features effectively, we used the MoSCoW method, categorizing features into Must-have, Should-have, Could-have, and Won’t-have. This approach helped us focus on essential functionalities, such as:
- Task Management: Allow users to create, edit, and assign tasks.
- Team Collaboration: Enable real-time updates and communication among team members.
- Progress Tracking: Provide visual indicators of project progress to keep teams aligned.
- User Authentication: Implement secure login and registration to protect user data.
By defining these core features, we ensured that the MVP would address key user needs while remaining focused and achievable.
4. Designing the User Interface and User Experience
A seamless and intuitive user experience was a top priority for this project. We aimed to design an interface that was not only visually appealing but also easy to navigate, with a clean and minimalistic layout.
Wireframing and Prototyping
We started by creating wireframes to visualize the user flow and layout. Using tools like Figma, we developed low-fidelity prototypes to outline the main sections of the application, such as the dashboard, task creation page, and team collaboration interface. Prototyping helped identify potential usability issues early, allowing us to refine the design before moving into development.
Responsive Design
Given the increasing use of mobile devices for work-related tasks, we ensured that the design was fully responsive. By using a mobile-first approach, we created layouts that adapted seamlessly to different screen sizes, providing a consistent experience across devices.
5. Setting Up the Development Environment
A well-organized development environment is essential for productivity and collaboration. For this project, we set up a development environment that included key tools and configurations to streamline coding, testing, and deployment.
Version Control and Collaboration
We used Git and GitHub for version control, enabling team members to collaborate efficiently and track changes. Git branches were set up for each feature, making it easier to manage updates and conduct code reviews.
Configuring the Next.js Project
We initialized the Next.js project with the following key configurations:
- TypeScript: Adding TypeScript provided static typing, helping to catch errors early and improve code reliability.
- ESLint and Prettier: We configured ESLint and Prettier to enforce code quality and consistency, ensuring that the codebase remained clean and readable.
- Styled Components: Styled Components allowed us to manage CSS within JavaScript, making it easier to style components dynamically based on props and state.
Setting up these tools and configurations early in the process helped establish a productive workflow and minimize technical debt.
6. Building the Foundation: Key Components and Layout
With the development environment ready, we began building the foundational components of the application. This stage focused on creating reusable, modular components that would serve as building blocks for the MVP.
Header and Navigation Components
The header and navigation bar were essential for providing seamless access to different sections of the application. We designed a responsive navigation bar with links to the dashboard, tasks, team, and settings. By using conditional rendering, we ensured that only authenticated users could access certain routes, enhancing security.
Task Card Component
The task card was one of the core components of the MVP, displaying information about each task, such as title, assigned team members, and due date. We used props to make the component dynamic, allowing it to display different content based on the task data passed to it.
import React from "react";
import styled from "styled-components";
const TaskCard = ({ title, assignee, dueDate }) => (
<Card>
<h3>{title}</h3>
<p>Assigned to: {assignee}</p>
<p>Due: {dueDate}</p>
</Card>
);
const Card = styled.div`
padding: 1rem;
background-color: #f9f9f9;
border-radius: 5px;
margin-bottom: 1rem;
`;
export default TaskCard;
By designing modular components, we ensured that each piece of the interface could be reused and modified independently, simplifying future updates.
7. Implementing User Authentication
User authentication was critical for ensuring that only authorized users could access the platform. We implemented authentication using JWT (JSON Web Tokens) and set up a secure login and registration system.
Using Firebase Authentication
For simplicity and scalability, we chose Firebase Authentication to manage user registration, login, and authentication states. Firebase provided a secure, easy-to-integrate solution that saved development time and allowed us to focus on other core features.
Protecting Routes with Authentication Middleware
To secure private routes, we used middleware to check if users were authenticated before granting access. This approach ensured that non-authenticated users were redirected to the login page, protecting sensitive data and enhancing security.
8. Integrating Data Storage with Firebase
For this MVP, we used Firebase Firestore as the primary database. Firestore’s real-time data capabilities and flexibility made it an ideal choice for managing task data and user information.
Setting Up Firestore Collections
We created collections for tasks, users, and project data, organizing the database to support efficient data retrieval and updates. Firestore’s NoSQL structure allowed us to model the data in a way that aligned with the app’s structure, making it easy to manage relationships between tasks, users, and projects.
Real-Time Data Synchronization
One of the advantages of Firestore is real-time data synchronization. By leveraging this feature, we enabled real-time updates for task assignments and status changes, allowing team members to see updates immediately without refreshing the page.
Conclusion and Next Steps
This first part of the case study covered the initial stages of building a SaaS MVP with React and Next.js, including planning, design, and the development of foundational components. In Part 2, we’ll dive deeper into implementing advanced features, optimizing performance, and preparing the MVP for deployment.
Creating a SaaS MVP requires a methodical approach, balancing the need for essential functionality with a focus on scalability and user experience. By leveraging React and Next.js, we were able to create a high-performance, scalable application that met the project’s core objectives.
Stay tuned for Part 2, where we’ll continue exploring the development process and share more insights into building a successful SaaS MVP.