Case Study: Building a SaaS MVP with React and Next.js (Part 2)
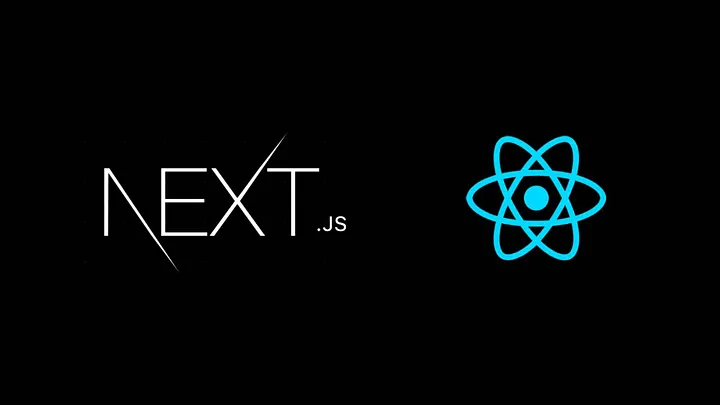
In the first part of our case study, we explored the initial stages of building a SaaS MVP with React and Next.js, covering ideation, planning, and foundational development. In Part 2, we’ll dive into advanced features, performance optimization, deployment strategies, and the lessons learned. This phase focuses on enhancing functionality, ensuring scalability, and preparing the MVP for market launch.
1. Implementing Advanced Features and Enhancements
With the core functionalities in place, the next step involved adding advanced features to enhance usability and value. These features aimed to provide additional utility to users while maintaining a simple, intuitive experience.
Task Comments and Collaboration
To facilitate team collaboration, we added a comment section within each task. This allowed team members to communicate, share updates, and discuss details directly on the platform.
Technical Implementation
We created a new Comments
component with its own data structure, stored in Firestore. This component supported real-time updates, so users could see new comments without refreshing the page.
import React, { useState } from "react";
const Comments = ({ taskId }) => {
const [comments, setComments] = useState([]);
// Function to fetch and display comments
const fetchComments = async () => {
// Firebase Firestore fetch logic
};
return (
<div>
<h4>Comments</h4>
{comments.map((comment) => (
<p key={comment.id}>{comment.text}</p>
))}
</div>
);
};
export default Comments;
By integrating comments, we created a more interactive experience that improved teamwork and streamlined communication.
Notification System
To keep users informed about project updates, we implemented a notification system. This feature alerted users to new task assignments, comments, and status changes.
Real-Time Notifications with Firebase
Using Firebase’s real-time capabilities, we created a notification structure that instantly updated users whenever relevant changes occurred. Each user received a custom notification feed accessible from the dashboard, keeping them informed and engaged.
2. Performance Optimization Strategies
Optimizing performance was a key priority to ensure smooth operation, especially as the user base grew. We focused on strategies to reduce load times, enhance responsiveness, and improve Core Web Vitals.
###Optimizing Component Rerenders Excessive rerenders can slow down React applications, particularly in complex components. We utilized React’s memo function and the useMemo hook to prevent unnecessary rerenders, especially in high-usage components like the task list.
import React, { memo } from "react";
const TaskList = memo(({ tasks }) => (
<ul>
{tasks.map((task) => (
<li key={task.id}>{task.title}</li>
))}
</ul>
));
export default TaskList;
By optimizing rerenders, we improved performance and reduced CPU usage, resulting in a smoother user experience.
Lazy Loading and Code Splitting
To reduce the initial load time, we implemented lazy loading for components that were not immediately visible, such as the settings and profile pages. Additionally, we used code splitting to divide the JavaScript bundle, ensuring that users only loaded essential code on the initial page view.
Image Optimization
We used Next.js’s Image component to serve optimized images. This component automatically resizes and compresses images, improving load times, especially on mobile devices.
import Image from "next/image";
const ProfilePicture = () => (
<Image src="/profile.jpg" alt="Profile Picture" width={200} height={200} />
);
export default ProfilePicture;
By optimizing images, we improved page speed and enhanced the user experience, particularly for mobile users.
3. Testing and Quality Assurance
Testing was an essential part of the development process to ensure that the MVP met performance and usability standards. Our approach included functional, usability, and load testing.
Functional Testing
We conducted functional tests for all core features, including task management, comments, and notifications. Automated testing tools like Jest and React Testing Library helped ensure that each component behaved as expected.
Usability Testing
Usability testing allowed us to gather feedback on the MVP’s user interface and overall experience. We invited a sample group of users to interact with the application and provide feedback on ease of use, navigation, and design elements. This feedback helped us identify areas for improvement and make necessary adjustments.
Load Testing for Scalability
To prepare for a larger user base, we conducted load testing to assess the application’s performance under simulated high-traffic conditions. This testing helped us identify potential bottlenecks, ensuring that the MVP remained responsive even with a high number of concurrent users.
4. Preparing for Deployment
With development complete, the final step was deploying the MVP. We focused on selecting a hosting solution, configuring environment variables, and setting up a continuous deployment pipeline.
Choosing the Right Hosting Environment
We selected Vercel for hosting due to its seamless integration with Next.js, automated deployments, and scalability. Vercel’s serverless infrastructure allowed us to deploy the MVP without managing physical servers, simplifying maintenance.
Setting Up Environment Variables
To keep sensitive information secure, we configured environment variables for keys and API endpoints. This approach enhanced security and made it easier to manage configurations across different environments, such as staging and production.
Continuous Deployment with GitHub Actions
To streamline future updates, we set up a continuous deployment pipeline using GitHub Actions. This automation ensured that any changes pushed to the main branch triggered a new deployment, keeping the MVP up-to-date without manual intervention.
name: Deploy to Vercel
on:
push:
branches:
- main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- run: npm install
- run: npm run build
- run: vercel --prod
This setup allowed us to maintain an efficient workflow, facilitating quick updates and ensuring that the latest features and fixes were always live.
5. Launch and Post-Launch Optimization
After deploying the MVP, we monitored performance and gathered user feedback to refine the product. The launch was an opportunity to validate our work and identify areas for improvement based on real-world usage.
Monitoring Performance
We used Google Analytics and Firebase Performance Monitoring to track user interactions, load times, and other key metrics. This data provided insights into user behavior, highlighting areas for potential enhancements.
Gathering User Feedback
User feedback was invaluable for understanding how the MVP met user needs and identifying areas for further development. Based on feedback, we prioritized a list of features and improvements for future updates, including additional integrations, advanced reporting, and customization options.
6. Lessons Learned and Key Takeaways
Building a SaaS MVP with React and Next.js was a valuable learning experience, offering insights into effective project management, development strategies, and user-centered design.
Importance of Modular Components
Creating modular, reusable components saved development time and facilitated scalability. By building with reuse in mind, we could extend the MVP without extensive rewrites, allowing for faster iteration in future updates.
Balancing Features and Performance
One of the biggest challenges was balancing feature richness with performance. By focusing on core functionality and using optimization techniques, we created an MVP that provided value to users without compromising speed or responsiveness.
Value of Real-Time Data
Real-time data capabilities, especially for comments and notifications, significantly improved user engagement. Users appreciated the immediacy of real-time updates, reinforcing the importance of responsiveness in SaaS applications.
Conclusion: Building a Scalable, User-Centric SaaS MVP
This case study highlights the step-by-step process of building a SaaS MVP with React and Next.js, from planning and initial development to advanced features, testing, and deployment. By focusing on modularity, performance, and user experience, we successfully created an MVP that met core objectives and provided a foundation for future growth.
Whether you’re planning to build a new SaaS product or refine an existing platform, Prompt Launch can help turn your vision into a functional, scalable MVP. Contact Prompt Launch to explore how we can bring your SaaS project to life with a tailored, efficient approach.