Modern Web Architecture Patterns for Next-Level SaaS Applications
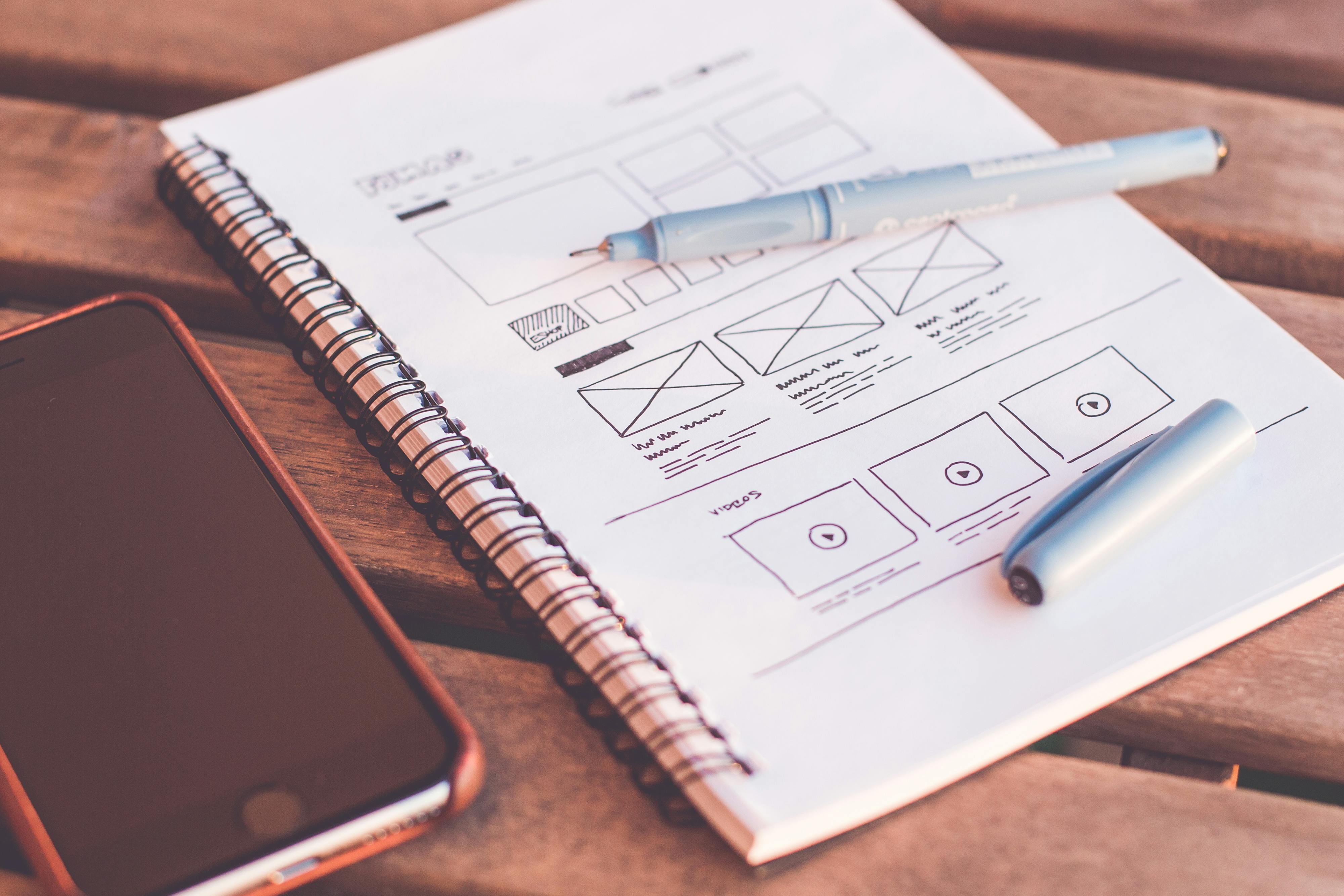
Introduction
Software as a Service (SaaS) has revolutionized the landscape of modern business operations, providing a scalable and cost-effective alternative to on-premise software. As the SaaS market continues to flourish, it's imperative to stay abreast of the latest web architecture patterns that can help your application stay competitive and scalable.
In this blog post, we will delve deep into the modern web architecture patterns that are shaping the SaaS industry. We'll provide practical examples, code snippets, and actionable takeaways to help you design and build your next-generation SaaS applications.
Microservices Architecture
Microservices architecture is an architectural style that structures an application as a collection of loosely coupled, independently deployable services. Each microservice runs a unique process and communicates through a well-defined, lightweight mechanism, typically an HTTP/HTTPS-based API.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "Hello World from Flask Microservice!"
This architecture provides several benefits for SaaS applications:
- Scalability: Each microservice can be scaled independently based on demand.
- Faster Time to Market: Teams can work on different services simultaneously, speeding up development.
- Resilience: If one microservice fails, it doesn’t affect the functioning of others.
Serverless Architecture
Serverless architecture is a software design model where a third-party service hosts and executes the application's server-side logic and state.
// A simple AWS Lambda function
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify("Hello from Lambda!"),
};
return response;
};
Serverless architecture offers the following advantages for SaaS applications:
- Cost Efficiency: You only pay for the computing resources you use.
- Scalability: Your application can seamlessly scale up or down based on demand.
- Productivity: Developers can focus on the app's core functionality instead of managing servers.
Event-Driven Architecture
Event-Driven Architecture (EDA) is a design pattern where the flow of the program is determined by events such as sensor outputs, user actions, or messages from other programs.
document.addEventListener("click", function () {
console.log("An event occurred!");
});
EDA offers several benefits for SaaS applications:
- Responsiveness: EDA can facilitate real-time updates and responsiveness.
- Scalability: It can handle high traffic and heavy load.
- Loose Coupling: Components interact only through events, leading to a flexible and adaptable system.
API-First Architecture
API-first architecture is a design approach where APIs are treated as first-class citizens. The API is designed and built before the application itself.
// Express.js API Endpoint
app.get("/api/v1/users", function (req, res) {
res.send("Fetch all users");
});
API-first architecture has the following advantages:
- Consistency: It ensures a consistent experience across multiple devices and platforms.
- Efficiency: It allows different teams to work simultaneously on the frontend and backend.
- Extensibility: Other developers can use your API to build their own apps.
Conclusion
In the fast-paced SaaS landscape, adopting the right web architecture pattern is crucial to building scalable, efficient, and robust applications. Microservices, Serverless, Event-Driven, and API-first Architectures are some of the modern patterns that can help you stay ahead of the curve.
Remember, there's no one-size-fits-all solution. The choice of architecture depends on your specific use-case, team expertise, and business goals. Therefore, always evaluate the pros and cons of each architecture pattern before making a decision.
In the upcoming blog posts, we will delve deeper into each architecture style, exploring their best practices, anti-patterns, and real-world implementations. Stay tuned!