Mastering Progressive Web Apps: Implementation Strategies for Modern Web Development
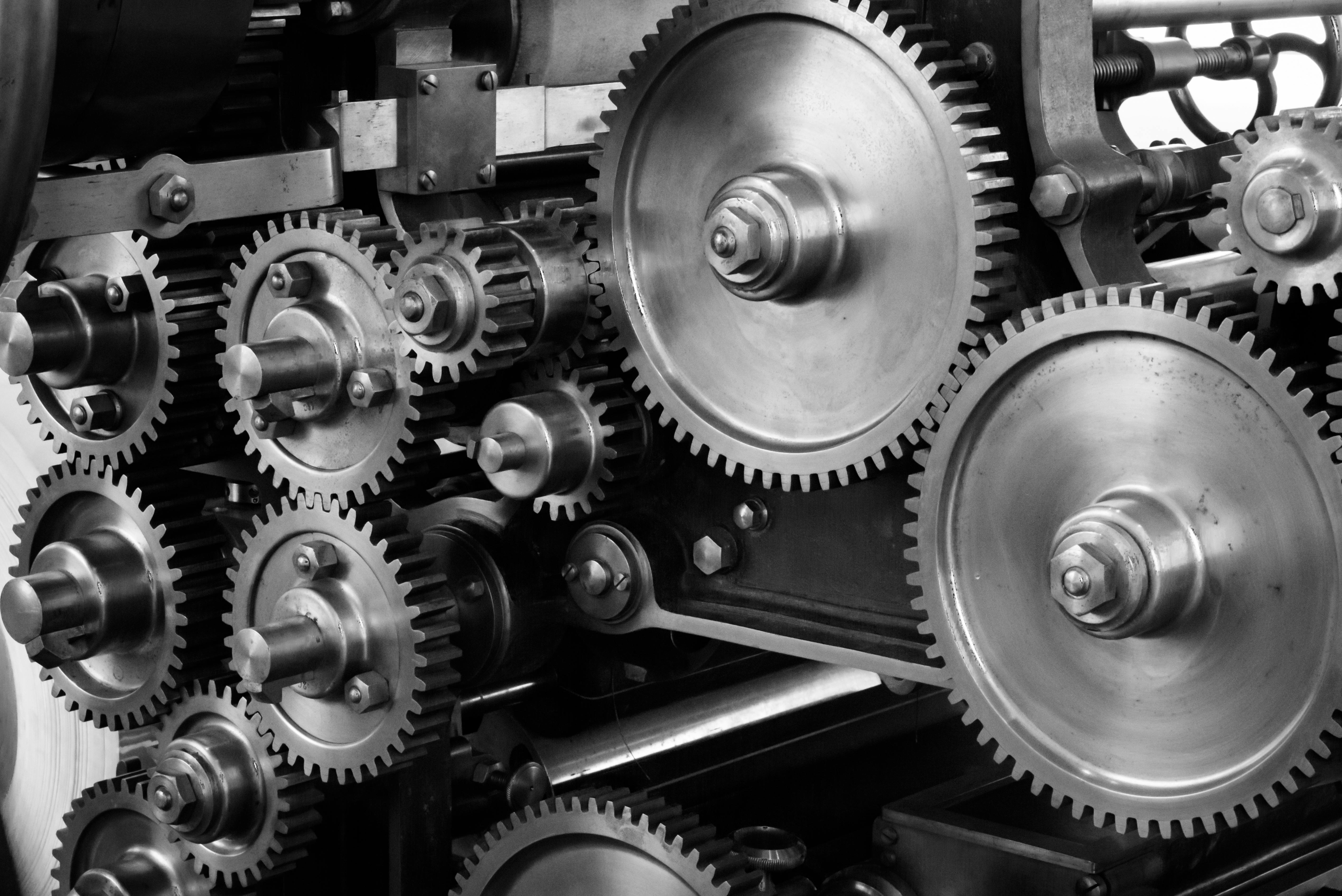
Mastering Progressive Web Apps: Implementation Strategies for Modern Web Development
In today's fast-paced digital world, Progressive Web Apps (PWAs) have emerged as a groundbreaking approach to deliver seamless and engaging web experiences. By bridging the gap between web and mobile applications, PWAs offer numerous advantages such as improved performance, offline capabilities, and enhanced user engagement. This comprehensive guide explores effective PWA implementation strategies, providing practical examples and code snippets to help developers create state-of-the-art applications.
Understanding Progressive Web Apps
Progressive Web Apps are web applications that use modern web technologies to deliver app-like experiences on the web. They are designed to work on any platform that uses a standards-compliant browser, including both desktop and mobile devices. PWAs leverage features like service workers, web app manifests, and responsive design to offer fast, reliable, and engaging user experiences.
Key Features of PWAs
- Responsiveness: PWAs adapt seamlessly to different screen sizes and orientations, ensuring a consistent user experience across devices.
- Offline Functionality: Through service workers, PWAs can cache resources and enable offline access, providing uninterrupted experiences even with poor or no internet connectivity.
- App-like Interactions: PWAs can be installed on a user's home screen, offering quick access and a native app-like feel.
- Push Notifications: PWAs support push notifications, allowing timely and relevant communication with users.
- Secure: PWAs are served over HTTPS, ensuring secure data exchange between the client and server.
Core PWA Implementation Strategies
Implementing a Progressive Web App involves several key steps and strategies. This section outlines the core components necessary for building a successful PWA.
1. Implementing Service Workers
Service workers are the backbone of a PWA, enabling offline functionality, background synchronization, and push notifications. They act as a proxy between the network and the application, intercepting network requests and handling caching.
Setting Up a Service Worker
To set up a service worker, you'll need to create a JavaScript file and register it in your application:
// Registering a service worker
if ("serviceWorker" in navigator) {
navigator.serviceWorker
.register("/service-worker.js")
.then((registration) => {
console.log(
"Service Worker registered with scope:",
registration.scope
);
})
.catch((error) => {
console.error("Service Worker registration failed:", error);
});
}
Caching Strategies
Caching strategies dictate how a service worker manages network requests and cached resources. Common caching strategies include:
- Cache First: Serve resources from the cache first, falling back to the network if not available in the cache. Ideal for frequently accessed assets like stylesheets and scripts.
self.addEventListener("fetch", (event) => {
event.respondWith(
caches.match(event.request).then((cachedResponse) => {
return cachedResponse || fetch(event.request);
})
);
});
- Network First: Try fetching the resource from the network first, falling back to the cache if the network is unavailable. Useful for dynamic content like API responses.
self.addEventListener("fetch", (event) => {
event.respondWith(
fetch(event.request).catch(() => caches.match(event.request))
);
});
2. Creating a Web App Manifest
The web app manifest is a JSON file that provides metadata for your PWA, such as the app's name, icons, theme colors, and display properties. It enables the "Add to Home Screen" feature, allowing users to install the app on their devices.
Example Web App Manifest
{
"name": "My Progressive Web App",
"short_name": "MyPWA",
"start_url": "/index.html",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#3E4EB8",
"icons": [
{
"src": "/icons/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "/icons/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
3. Ensuring Responsiveness
A responsive design is crucial for a successful PWA, as it ensures the application looks and functions well on any device. Use CSS media queries and flexible layouts to achieve responsiveness.
Example Responsive Design
/* Mobile styles */
body {
font-size: 16px;
padding: 10px;
}
@media (min-width: 600px) {
/* Tablet styles */
body {
font-size: 18px;
padding: 20px;
}
}
@media (min-width: 1024px) {
/* Desktop styles */
body {
font-size: 20px;
padding: 30px;
}
}
Advanced PWA Implementation Strategies
Beyond the core components, several advanced strategies can enhance your PWA's performance, security, and user engagement.
1. Optimizing Performance
Optimizing performance is essential for retaining users and providing a smooth experience. Techniques like lazy loading, code splitting, and image optimization can significantly improve load times.
Lazy Loading
Lazy loading defers the loading of non-essential resources until they are needed, reducing initial page load time.
// Lazy loading an image
const img = document.createElement("img");
img.src = ""; // Placeholder source
img.dataset.src = "path/to/image.jpg";
img.className = "lazyload";
document.addEventListener("DOMContentLoaded", () => {
const lazyImages = document.querySelectorAll(".lazyload");
lazyImages.forEach((img) => {
img.src = img.dataset.src;
});
});
2. Enhancing Security
Security is paramount for PWAs, especially when handling sensitive user data. Ensure that your PWA is served over HTTPS and implement additional security measures like Content Security Policy (CSP) and Cross-Origin Resource Sharing (CORS).
Content Security Policy
CSP helps prevent cross-site scripting (XSS) attacks by specifying allowed sources for content.
Content-Security-Policy: default-src 'self'; script-src 'self'; img-src 'self' https://trustedsource.com;
3. Boosting User Engagement
Engagement features like push notifications and background synchronization can keep users actively involved with your PWA.
Push Notifications
Push notifications allow you to send timely updates to users, even when the app is not actively used.
// Requesting permission for notifications
Notification.requestPermission().then((permission) => {
if (permission === "granted") {
// Send a push notification
new Notification("Hello!", {
body: "Welcome to our Progressive Web App!",
icon: "/images/notification-icon.png",
});
}
});
Conclusion and Next Steps
Progressive Web Apps offer a powerful way to create engaging and reliable web experiences that rival those of native mobile applications. By implementing core features like service workers, web app manifests, and responsive design, and integrating advanced strategies for performance, security, and user engagement, developers can create state-of-the-art PWAs that meet modern user expectations.
As you embark on your PWA journey, consider the following next steps:
- Experiment with Different Caching Strategies: Test various caching strategies to find the best balance between performance and resource availability for your application.
- Leverage Modern Web APIs: Explore additional web APIs, such as the Web Share API or Payment Request API, to enhance your PWA's functionality.
- Continuously Optimize for Performance: Regularly assess and optimize your PWA's performance using tools like Lighthouse to ensure a smooth user experience.
By staying informed about the latest trends and best practices in PWA development, you can ensure your applications remain at the forefront of modern web technology.